Defining the web3 tech stack is more intricate than outlining traditional stacks like LAMP or MEAN. As the web3 development ecosystem continues to grow, new technologies and tools are also emerging at a rapid pace.
In this guide, we'll explore popular web3 development technologies that sit at the core of most Ethereum development projects.
Web3 tech stack overview
This guide covers the following components of the web3 tech stack:
- Ethereum development tools: Dive into the languages, libraries, and environments tailored for smart contract development.
- Blockchain networks: Understand the infrastructure that powers decentralized applications and smart contract execution.
- Node infrastructure services: Explore how Node as a Service providers enable access to the blockchain via RPC.
- Wallets: Discover the tools that enable users to interact with the blockchain, manage assets, and execute transactions.
- Data providers: Uncover the services that offer insights into on-chain activities and bring external data into the blockchain.
- Analytics and monitoring: Gain insights into on-chain activity related to transactions, contracts, and wallets.
- Decentralized storage: Explore the different solutions that enable data storage on decentralized systems.
Web3 development tools
Before starting work on any Ethereum-based project, you first need to decide on the tech stack that best fits your project's needs. Start with choosing a:
- Programming language for writing smart contracts
- Smart contract development framework
- Frontend library for interacting with deployed smart contracts
Smart contract programming languages
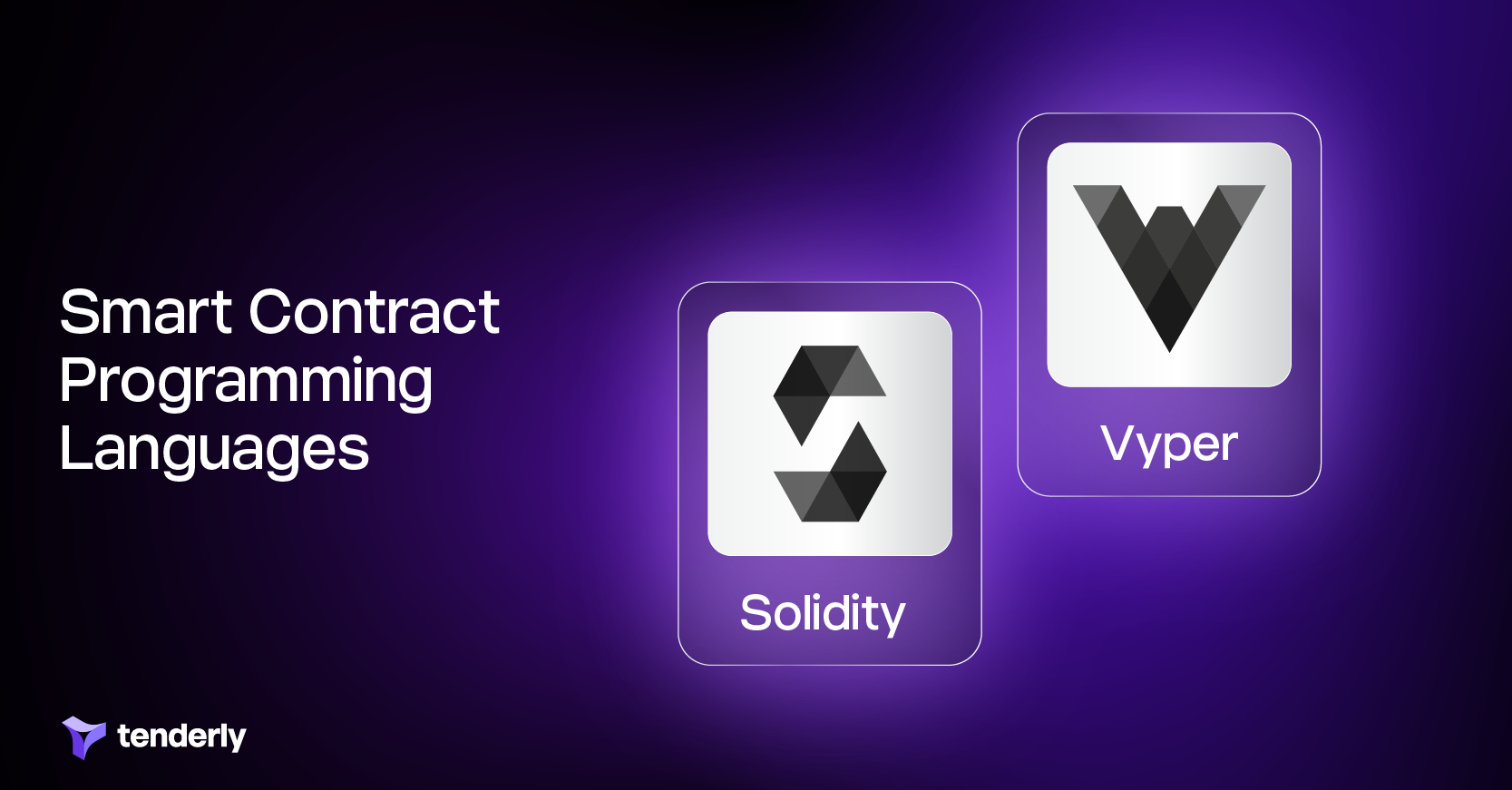
Two programming languages dominate the web3 tech stack: Solidity and Vyper.
Solidity is the de facto language for Ethereum smart contracts. Drawing inspiration from languages like JavaScript, Solidity is tailored for the Ethereum Virtual Machine (EVM). Its widespread adoption and robust community support make it the first choice for many developers.
Here's what a simple Solidity contract looks like:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
contract Counter {
uint public count;
// Function to get the current count
function get() public view returns (uint) {
return count;
}
// Function to increment count by 1
function inc() public {
count += 1;
}
// Function to decrement count by 1
function dec() public {
// This function will fail if count = 0
count -= 1;
}
}
On the other hand, Vyper has a Python-esque approach to smart contract development. It prioritizes security and simplicity. Vyper aims for clear, auditable code. It's a solid pick for projects with a strong security focus.
Here's how the same Solidity contract looks in Vyper:
# Counter contract in Vyper
count: public(uint256)
@public
@view
def get() -> uint256:
return self.count
@public
def inc() -> None:
self.count += 1
@public
def dec() -> None:
# This function will fail if count = 0
assert self.count > 0, "Count is already zero"
self.count -= 1
So, which language should you choose?
If you're gauging based on adoption, Solidity is your go-to language for writing smart contracts.
According to DefiLlama, Solidity dominates the DeFi space, securing 94% of TVL, while Vyper trails at 3%.
Differences between Solidity and Vyper
Solidity and Vyper are both high-level programming languages designed for writing smart contracts on Ethereum, but they differ in their design philosophies and features.
Solidity
- Overview: General-purpose, object-oriented smart contract language that can be used for a wide range of applications
- Popularity: Solidity is more widely used and has a larger community of developers.
- Complexity: It allows for more complex code, making it powerful but potentially riskier if not written correctly.
- Syntax: Solidity’s syntax is quite similar to JavaScript, making it somewhat familiar to people who have worked with JavaScript before.
- Features: It has more features (like inline assembly), which provide a lot of flexibility but can introduce more risks if used improperly.
- Tools: Since it's been around longer, it has more development tools and resources available.
Vyper
- Simplicity: Vyper aims to be more readable and simple, making it easier to audit the code.
- Security: It puts emphasis on security and is less permissive, meaning it doesn’t allow things that might make the code vulnerable (like inline assembly).
- Syntax: Vyper’s syntax is similar to Python, making it a good choice for developers familiar with Python.
- Features: Vyper deliberately has fewer features than Solidity to maintain simplicity and security.
- Tools: It has fewer tools and resources available compared to Solidity due to its younger age and smaller developer community.
Web3 frameworks and environments
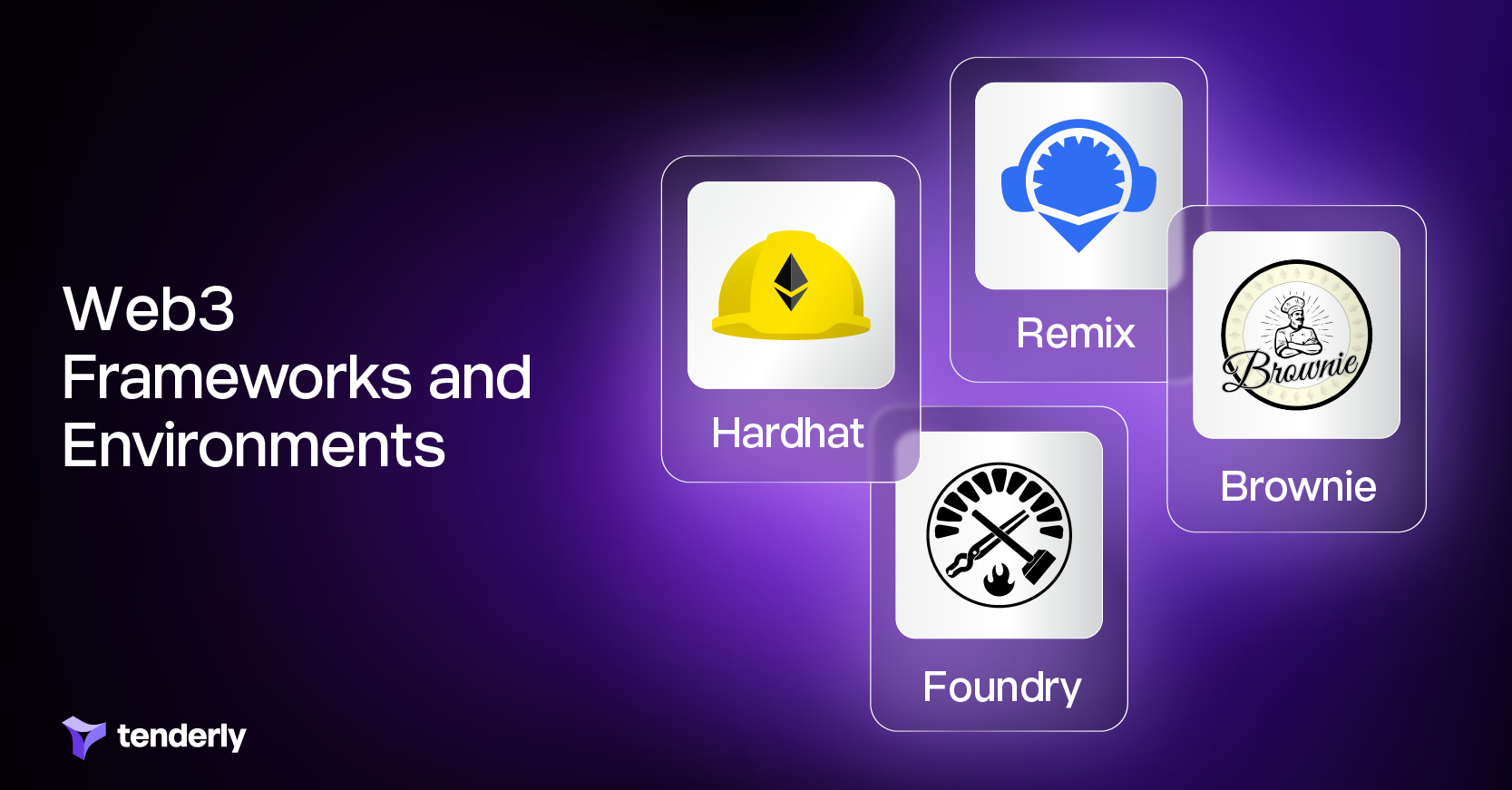
Once you've picked a language, the next step is to choose a smart contract development framework. These frameworks provide tools for the entire development lifecycle, from writing code to debugging and deployment.
The most used local development frameworks in web3 include:
Remix
Remix is Ethereum's official development suite. Its browser-based IDE supports both Solidity and Vyper. Remix is rich in features and a versatile choice for developers of all levels. It comes with a file explorer, code editor, console, Solidity compiler, and transaction debugger.
Whether you're accessing it online, via the Remix Desktop IDE, or as a VSCode extension, Remix is a reliable companion for any web3 developer.
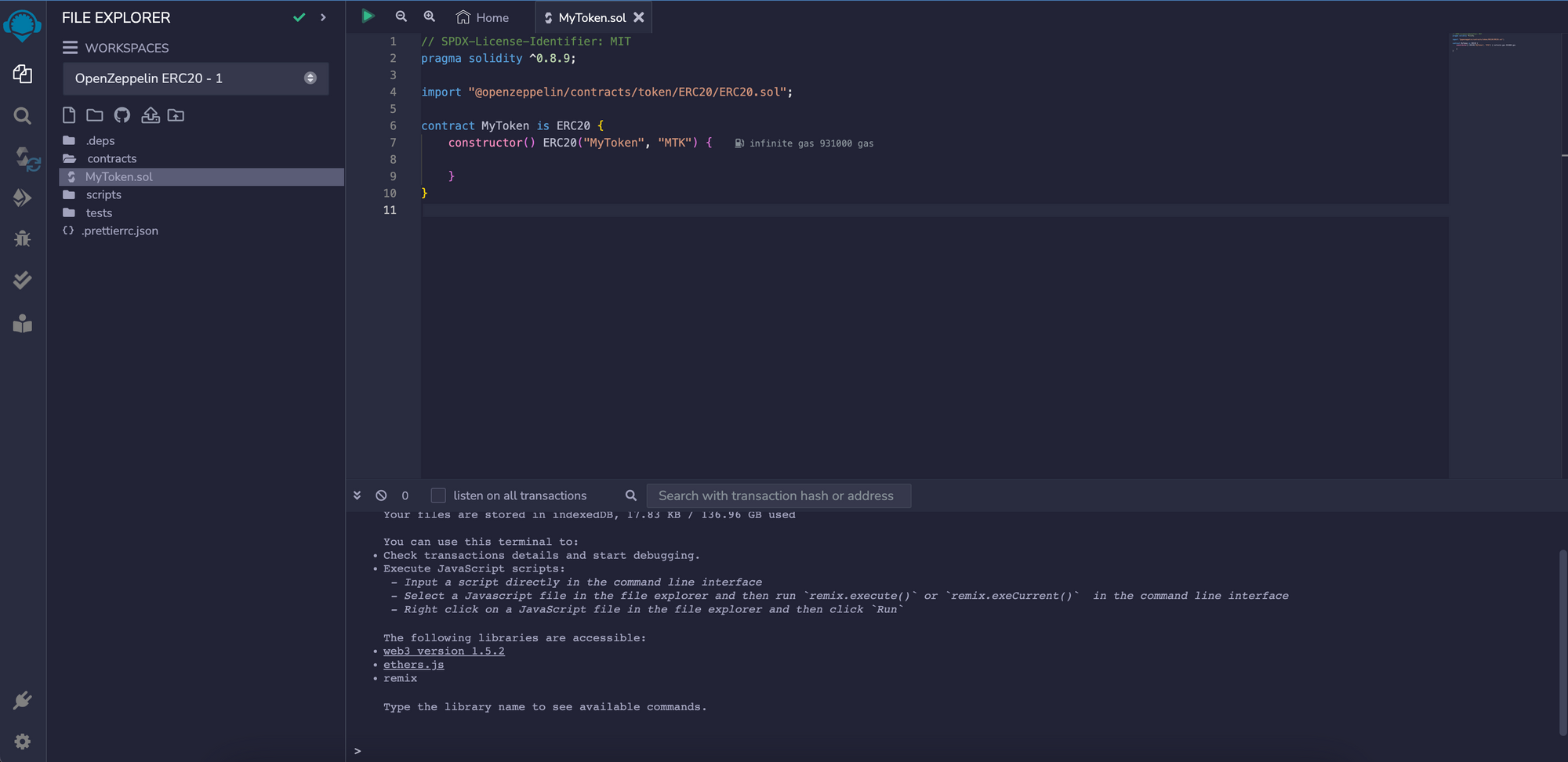
Hardhat
Hardhat is another heavyweight in the Ethereum development scene. It’s a JavaScript-based development environment.
You can install it using npm
or npx
.
# Install Harthat using npm
npm install --save-dev hardhat
# Start a new Hardhat project
npx hardhat init
Beyond its suite of development tools, Hardhat boasts a built-in local Ethereum node. This node is specially optimized for development, which can help streamline your testing and debugging workflows.
Hardhat makes it easy to deploy contracts locally, run tests, and debug code – without leaving your local environment.
However, you are not forced to use Hardhat’s local node. Hardhat allows you to add any network from any Node as a Service provider and run your transactions and contracts there.
For example, you can spin up a Tenderly DevNet and add the RPC URL to Hardhat. This will allow you to deploy contracts and execute transactions on a blockchain fork rather than on Hardhat's local node.
All you have to do is add the DevNet RPC URL to your Hardhat config file.
module.exports = {
networks: {
devnet: {
url: "https://rpc.vnet.tenderly.co/devnet/template-name/387db0-8809-...",
chainId: 1,
}
}
};
With DevNets, you get access to our visual Debugger and Transaction Simulator in local development. These tools make it much easier to inspect errors, function calls, gas usage, and the stack trace in great detail. Transaction Simulator comes in handy when you want to understand how your fixes will behave before deploying to production.
Foundry
Foundry is an all-in-one development environment tailored for Ethereum development. It's written in Rust.
While Hardhat is the most used framework, Foundry is steadily gaining traction with web3 developers.
The Foundry toolkit contains several components, including a testing framework. It offers tools for interacting with smart contracts, executing transactions, debugging, and retrieving blockchain data.
Similar to Hardhat, Foundry also ships with a local Ethereum node.
Foundry also works with Tenderly DevNets. Here’s a quick guide on how to integrate DevNets into your Foundry project.
Brownie
If you’re building in Python, Brownie is your development and testing framework for smart contracts.
It supports both Solidity and Vyper languages and offers debugging tools with tracebacks and custom error strings. You also get a built-in console for easier project management.
Install Brownie using pipx
or pip
.
pipx install eth-brownie
pip install eth-brownie
Brownie also comes with a GUI for viewing test coverage data and analyzing the compiled bytecode of your contracts.
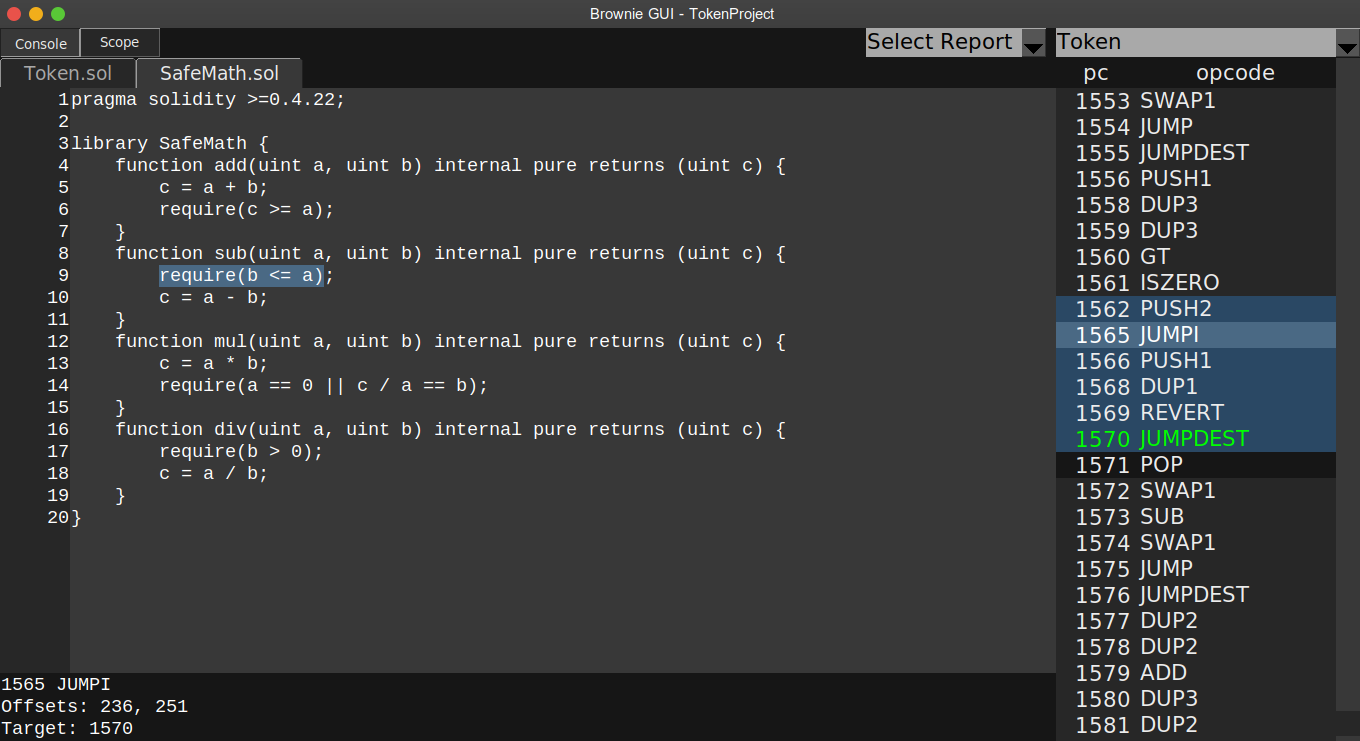
Truffle (Discontinued)
Truffle Suite is a popular end-to-end smart contract development framework. It enables you to compile, debug, and deploy smart contracts in one place. The toolkit also includes Ganache — a private blockchain for testing that runs on your local machine.
Even though Truffle was one of the go-to development frameworks, it was discontinued. Consensys announced that it was sunsetting Truffle, promising support until December 2023. Afterward, developers are advised to transition to Hardhat.
Web3 JavaScript and Python libraries
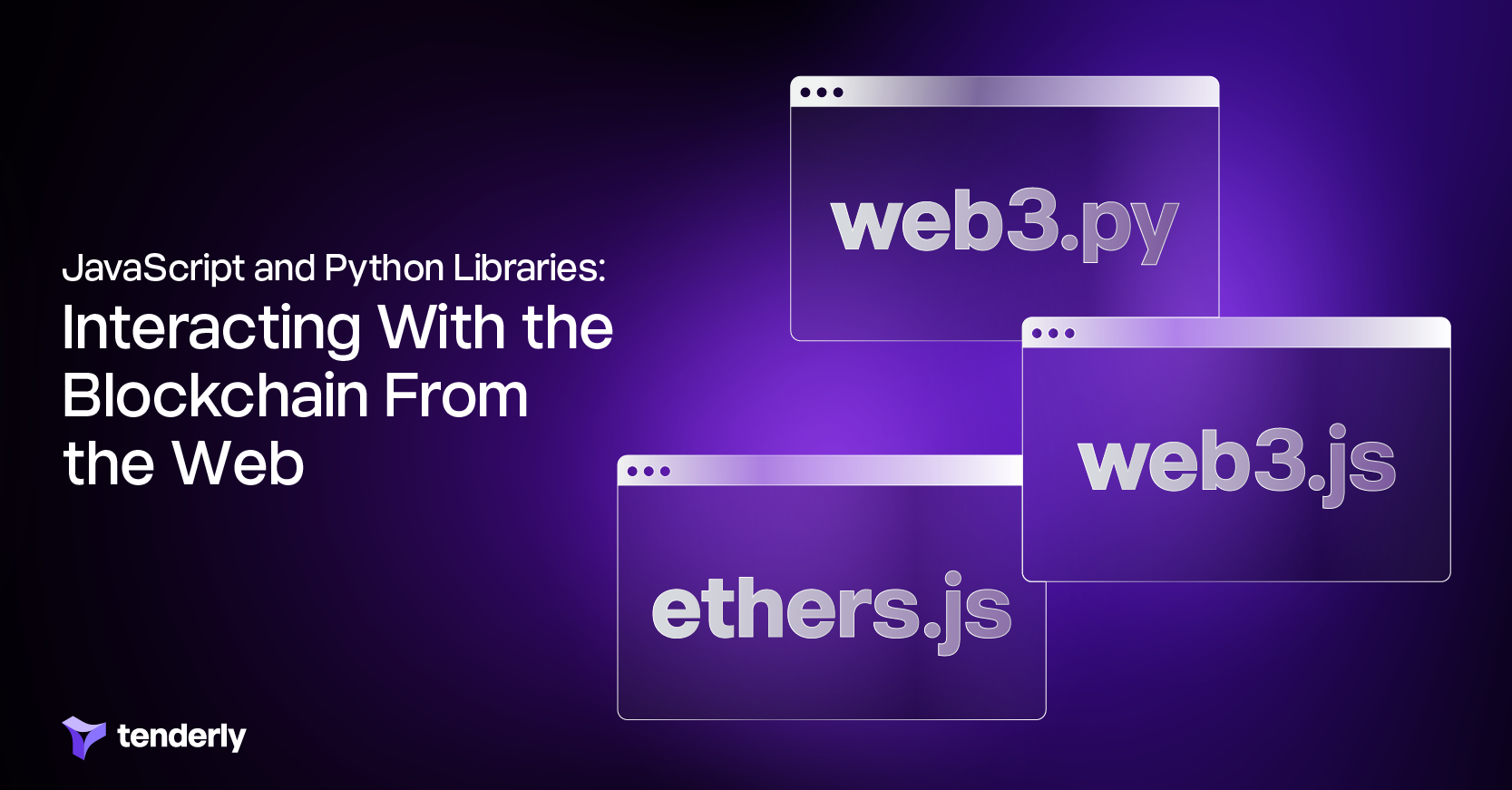
With your smart contract written and tested, the next step is to deploy it and interact with it on the live blockchain. For this, you'll need some sort of a bridge between your web application and the Ethereum blockchain.
Enter web3 libraries.
These libraries, whether in JavaScript or Python, handle tasks ranging from sending transactions to querying blockchain data. They're essential for integrating Ethereum functionalities into web applications or backend systems.
Choosing the right library for your project will depend on the language you are using and the level of control you want.
Here are the three most widely used JavaScript and Python libraries that are a must in any web3 tech stack. The example code snippets illustrate how each library works when extracting the latest block number from the Ethereum Mainnet.
web3.js
The official Ethereum JavaScript API, web3.js provides you with various libraries for interacting with local or remote Ethereum nodes via HTTP or WebSockets. It facilitates tasks such as sending transactions, querying balances, and deploying smart contracts.
// Import the web3.js library
const Web3 = require('web3');
// Create a new instance of Web3
const web3 = new Web3('https://mainnet.gateway.tenderly.co/YOUR_API_KEY');
// Access the web3 functionality
web3.eth.getBlockNumber().then(console.log);
ethers.js
Another popular open-source JavaScript library, ethers.js, offers a lightweight, optimized, and modular approach to perform Ethereum-related operations.
// Import the ethers.js library
const { ethers } = require('ethers');
// Create a node provider
const provider = new ethers.providers.JsonRpcProvider('https://mainnet.gateway.tenderly.co/YOUR_API_KEY');
// Query the current block number
provider.getBlockNumber().then((blockNumber) => {
console.log('Current Block Number:', blockNumber);
});
Web3.py
Web3.py is the Python counterpart to web3.js. It provides a suite of tools for Ethereum development in Python, making it easier to write, deploy, and interact with smart contracts.
# Import the web3.py library
from web3 import Web3
# Connect to a node provider
web3 = Web3(Web3.HTTPProvider('https://mainnet.gateway.tenderly.co/YOUR_API_KEY'))
# Get the current block number
block_number = web3.eth.block_number
print("Current Block Number:", block_number)
Differences between web3.js and ethers.js
web3.js
- Age & adoption: It's older and has been adopted by many developers for building dapps. It’s like the “old reliable” of Ethereum JavaScript libraries.
- Flexibility: web3.js provides a lot of flexibility and a wide range of functionality, which can be a bit overwhelming for new developers.
- Documentation: While it has extensive documentation, some developers feel it could be more clear and beginner-friendly.
- Community: web3.js has a large developer community, meaning a lot of support and resources are available.
- Size: The library itself is quite large, which might make your dapp heavier.
ethers.js
- Simplicity: ethers.js tends to prioritize simplicity and ease of use. It offers a clean and straightforward API.
- Documentation: It is often praised for having clear and well-organized documentation.
- Size: ethers.js is smaller and lighter than web3.js, which can result in lighter and faster dApps.
- Security: It's designed with security in mind and restricts some functionalities to avoid potential risks.
- Community: While it’s used by many, its community might be considered smaller compared to web3.js.
Blockchain networks
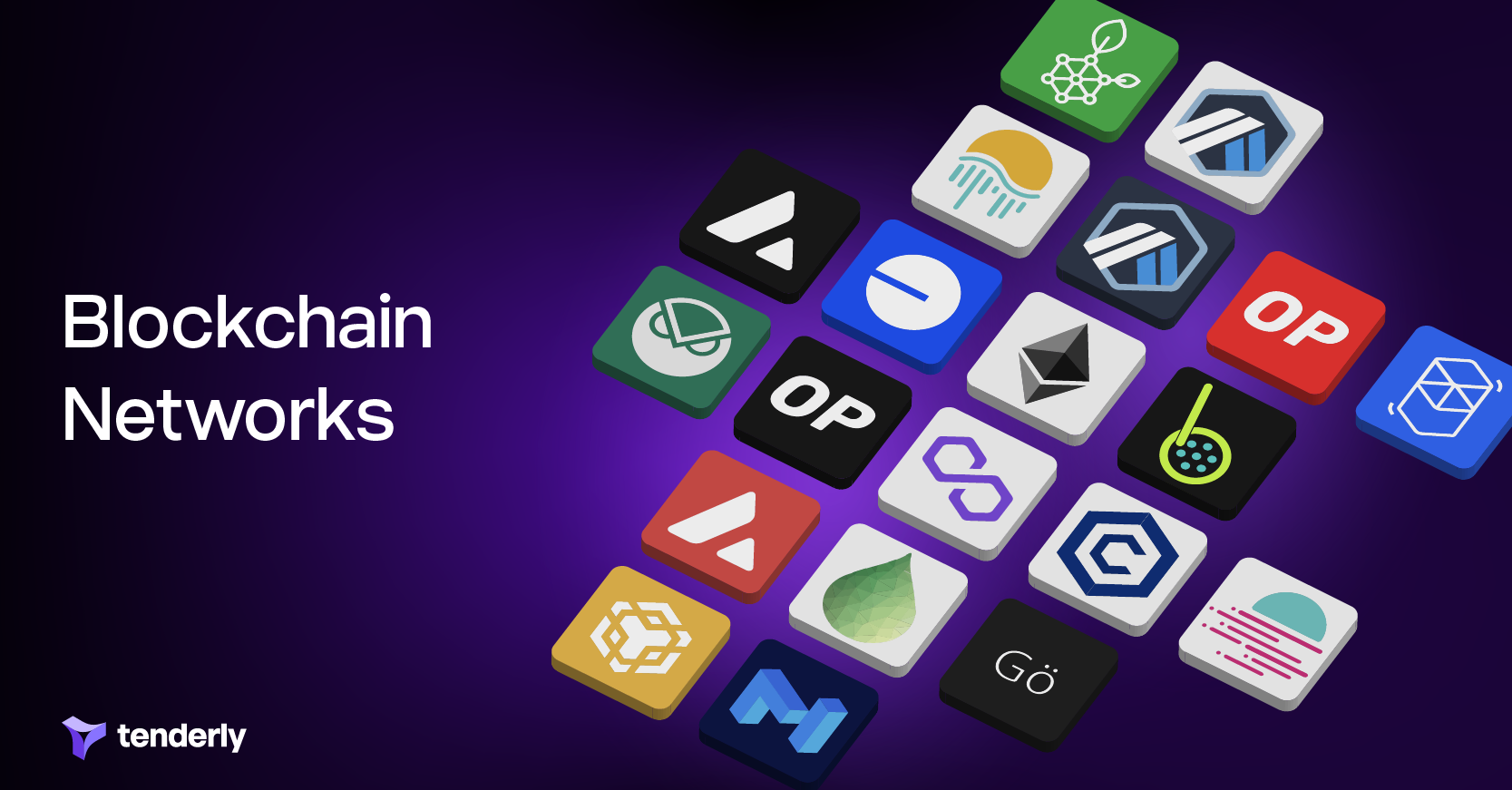
Blockchain networks serve as the execution layer for decentralized applications and smart contracts. There are multiple networks to choose from, and each offers unique options for various needs.
When selecting a network, consider which tools in your development tech stack are supported by the network. Also, take into account how you're going to test your dapps and the options each network offers. But in practice, you'll most likely be working with multiple chains simultaneously.
Mainnet: Production network
The Mainnet is where the action happens.
Often referred to as the production network, the Mainnet is the primary blockchain where real transactions take place. It's the live environment where cryptocurrencies have real value and smart contracts execute actual operations.
Engaging with the Mainnet means dealing with real stakes; operations here have real costs and are irreversible.
Layer 2 and rollups: Ethereum scaling solutions
Ethereum scaling solutions aim to increase the network's transaction throughput without compromising security. Known as Layer 2 network rollups, these solutions operate on top of the main Ethereum chain to process transactions more efficiently.
If you don’t want to deploy to the Ethereum Mainnet, you can choose to deploy on any of the more popular Layer 2 networks.
- Polygon: A mainstream choice for Layer 2 scaling.
- Arbitrum: Tailored for developers seeking efficient scaling.
- Optimism: Renowned for its seamless Ethereum compatibility.
- zkSync: A top pick for those emphasizing security.
Testnets: Testing networks
Before deploying to production networks, developers often use testing networks, or "testnets," to experiment and validate their smart contracts and dapps. These networks mimic the Mainnet's behavior but use valueless tokens, giving you a risk-free environment for testing.
The remaining Ethereum testnets currently operational are:
Layer 2 scaling solutions also have their own separate testnets. For example, Polygon has Mumbai, Arbitrum has the Arbitrum Goerli Testnet, and Optimism has the Optimism Goerli testnet.
While testnets are designed to mirror real blockchain conditions, they have limitations. The most obvious drawback is that they don't provide a real clone of the production data.
For serious and large-scale projects, testing with real production data is vital. This ensures your dapps are tested in an identical Mainnet environment.
If you're looking for such a solution – a short-lived dev and testing environment that runs on Mainnet data – Tenderly DevNets is the way to go.
Tenderly DevNets: Test and develop on production data
Tenderly DevNets allow you to deploy contracts, dapps, and execute transactions against a real-time replica of the Mainnet.
DevNets are customizable local blockchain forks that you can spin up on demand for testing and development purposes. They give you a temporary copy of the Ethereum Mainnet, testnets, or any of the Layer 2 blockchains.
Supporting over 30 networks, DevNets allow you to modify the network’s state and customize it any way you’d like.
Unlike public testnets, DevNets give you full control over the network's state, allowing for precise testing scenarios and faster iteration cycles.
What makes DevNets unique is that all the accounts on the fork are unlocked. This means you can change the funds on any contract or wallet to any value. You even get an unlimited faucet, so no more begging or mining for test ETH on public testnets.
How to spawn a DevNet RPC URL
In terms of integrations, DevNets fit seamlessly into your local projects. Adding DevNets into your Hardhat, Foundry, Brownie, or Truffle projects is as simple as adding an RPC URL to the config file.
In addition to local development, DevNets can also be set up to automatically execute tests on your contract code as part of your continuous integration pipeline. Setting up CI for your smart contract project with DevNets ensures that your code is always tested and verified before deployment.
Web3 nodes
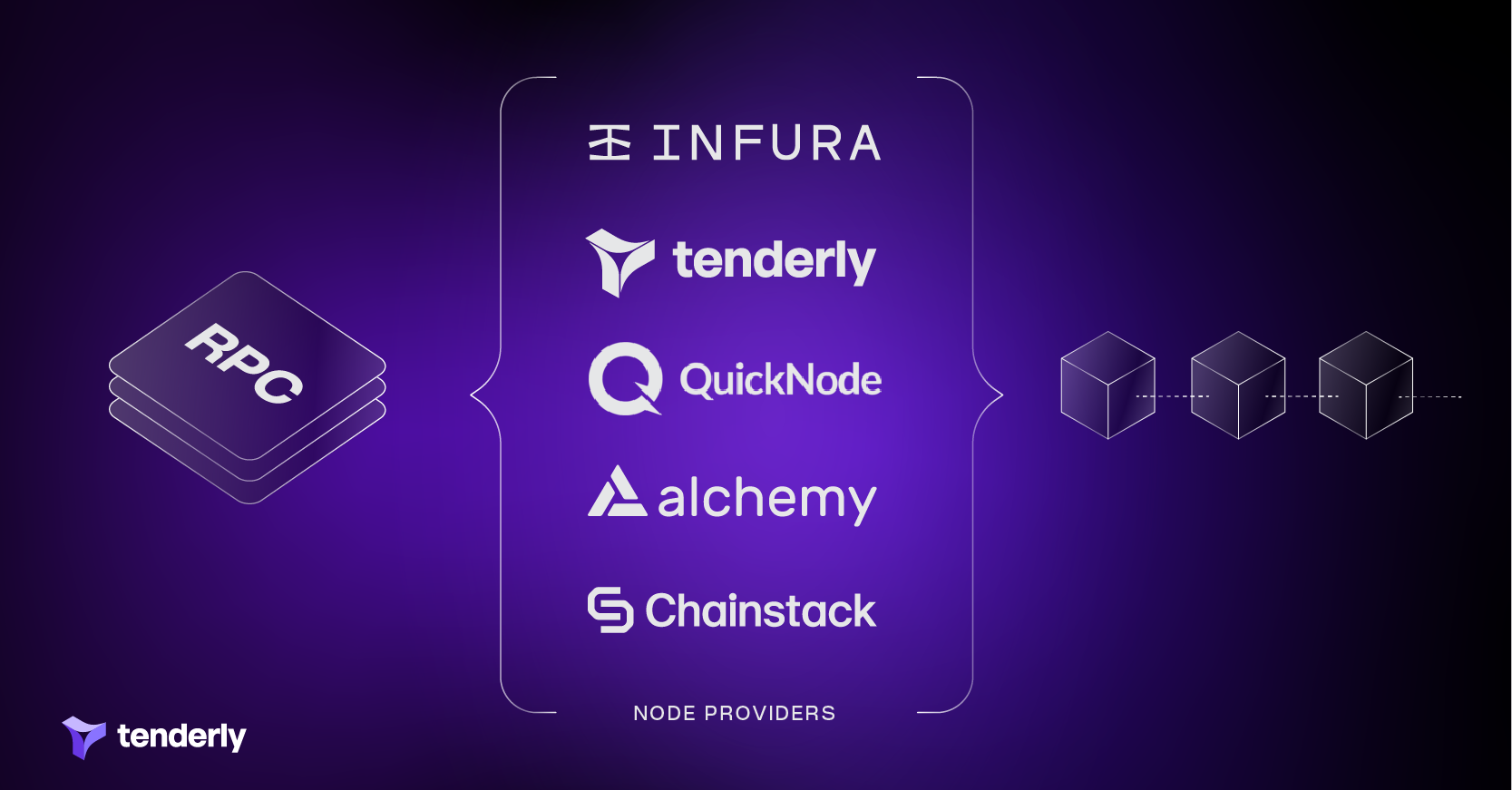
To deploy to the Ethereum Mainnet, you need to have access to a node. Developers usually don’t want to run and maintain their own nodes, so they use Node as a Service provider.
Node providers serve as the gateway to the blockchain. They simplify the process of connecting to blockchains, allowing you to focus on building apps rather than managing infrastructure.
Using simple RPC calls, you can perform actions such as querying transaction details, retrieving account balances, sending transactions, and interacting with smart contracts.
There are several Node as a Service providers available. Here are five standout providers that have earned acclaim for their reliability, robust features, and widespread adoption:
- Infura: One of the most popular node providers on the market.
- Tenderly Node: Reliable and performant production node integrated with Tenderly’s powerful suite of web3 developer tools.
- Alchemy Supernode: Unlimited requests to archive data, including forking the entire chain from Genesis.
- QuickNode: Fast and scalable node access to multiple chains.
- Chainstack: Global elastic full nodes with access to over 25 chains.
How to access the blockchain?
Tenderly Node provides a seamless interface for interacting with the blockchain via RPC.
To demonstrate, let’s get the latest block number on the Mainnet using the Ethers.js library and Tenderly Node.
- Go to Tenderly Node from the Dashboard and copy the RPC URL. The API key is part of the RPC URL.
- Add the URL as the RPC provider in Ethers.js.
- Call the
getBlockNumber()
method.
const { ethers } = require('ethers');
async function executeMethod() {
// Initialize an ethers instance
const provider = new ethers.providers.JsonRpcProvider('https://mainnet.gateway.tenderly.co/API_KEY');
// Execute method
const blockNumber = await provider.getBlockNumber();
// Print the output to console
console.log(blockNumber);
}
executeMethod();
Wallets
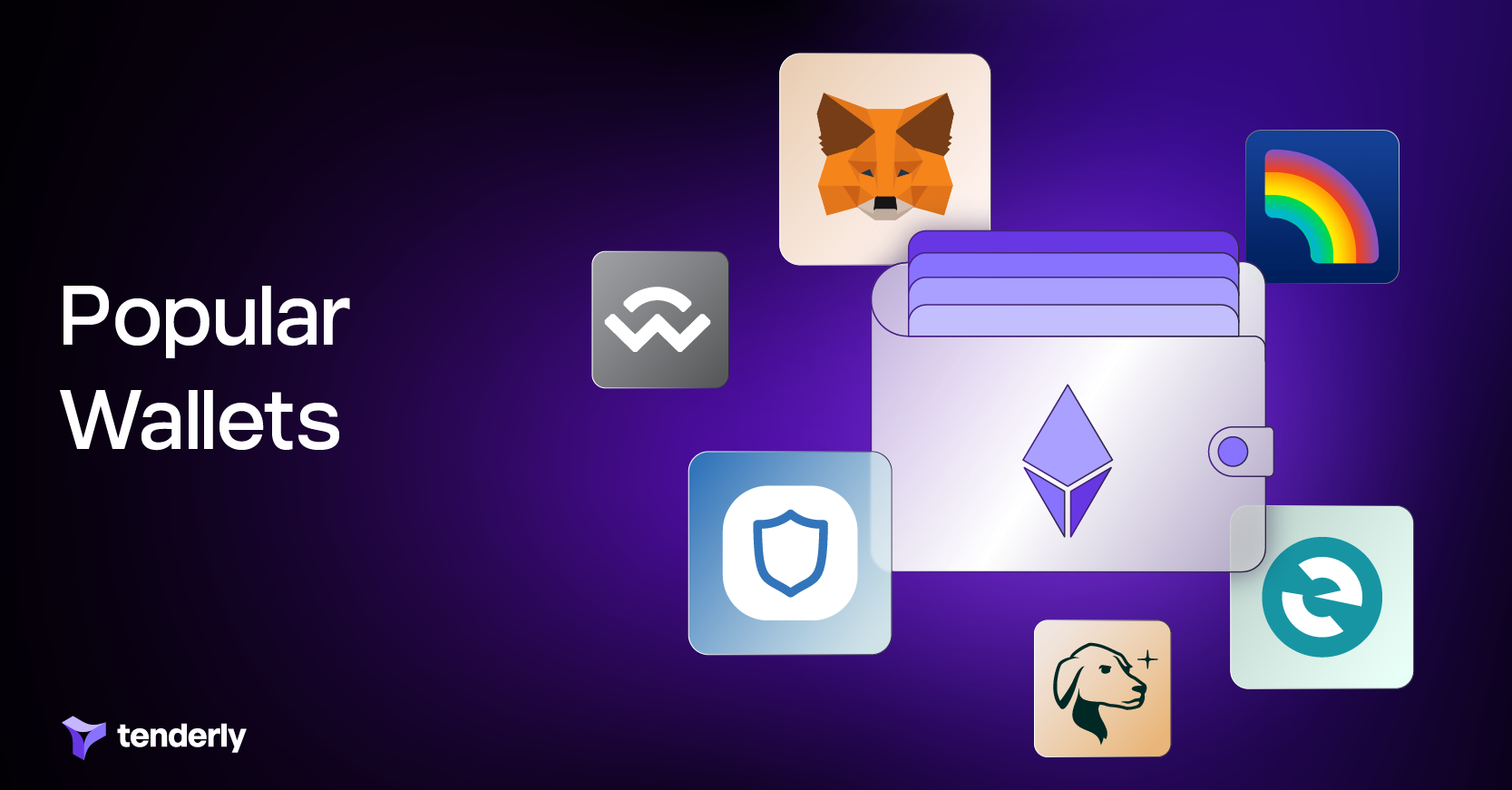
Digital wallets are fundamental components of the Ethereum ecosystem. They serve both as secure storage mechanisms for digital assets and as interfaces for smart contract interaction and dapp integration.
For developers, choosing the right wallet is crucial, not just for asset management but also for testing, deploying, and ensuring seamless user experiences.
Here's a deeper dive into several popular wallets tailored for developers:
- MetaMask: MetaMask is a browser extension that enables developers to send and receive tokens without running a full Ethereum node. Its ability to manage user identities and sign blockchain transactions makes it an invaluable tool for dapp development and testing. Developers can also use MetaMask Snaps to build on top of the wallet and expand its functionality.
- MyEtherWallet (MEW): A versatile, open-source, client-side interface, MEW provides developers with a suite of tools for creating and handling Ethereum transactions. Its flexibility allows for integration with various hardware wallets.
- Rabby Wallet: Rabby Wallet is an open-source, browser-extension wallet that supports over 95 blockchains. It prioritizes transaction security by scanning for potential risks and errors before the transaction is signed. Rabby Wallet also displays expected balance changes during transaction signing.
- Rainbow Wallet: Rainbow Wallet is a non-custodial mobile wallet for Ethereum, meaning users are in control of their private keys and funds. The wallet is also open source and has a sleek user interface that is easy to use. Rainbow Wallet has several features, including a built-in fiat on-ramp, WalletConnect integration, ENS support, NFT display support, and Uniswap integration.
- Taho Wallet (previously Tally Ho): Taho is a community-owned Web3 wallet that offers support for DeFi and NFTs. The wallet is 100% open source and self-custodial, giving users full control over their private keys and funds. The code running the wallet has been independently verified.
- WalletConnect: WalletConnect is an open-source developer toolkit that provides various SDKs and APIs for connecting wallets to dapps. It's also a communication protocol that standardizes the way wallets and dapps communicate. Some notable features include Dapp Messaging, Push Notifications for wallets, and Web3 Chat.
Blockchain data providers
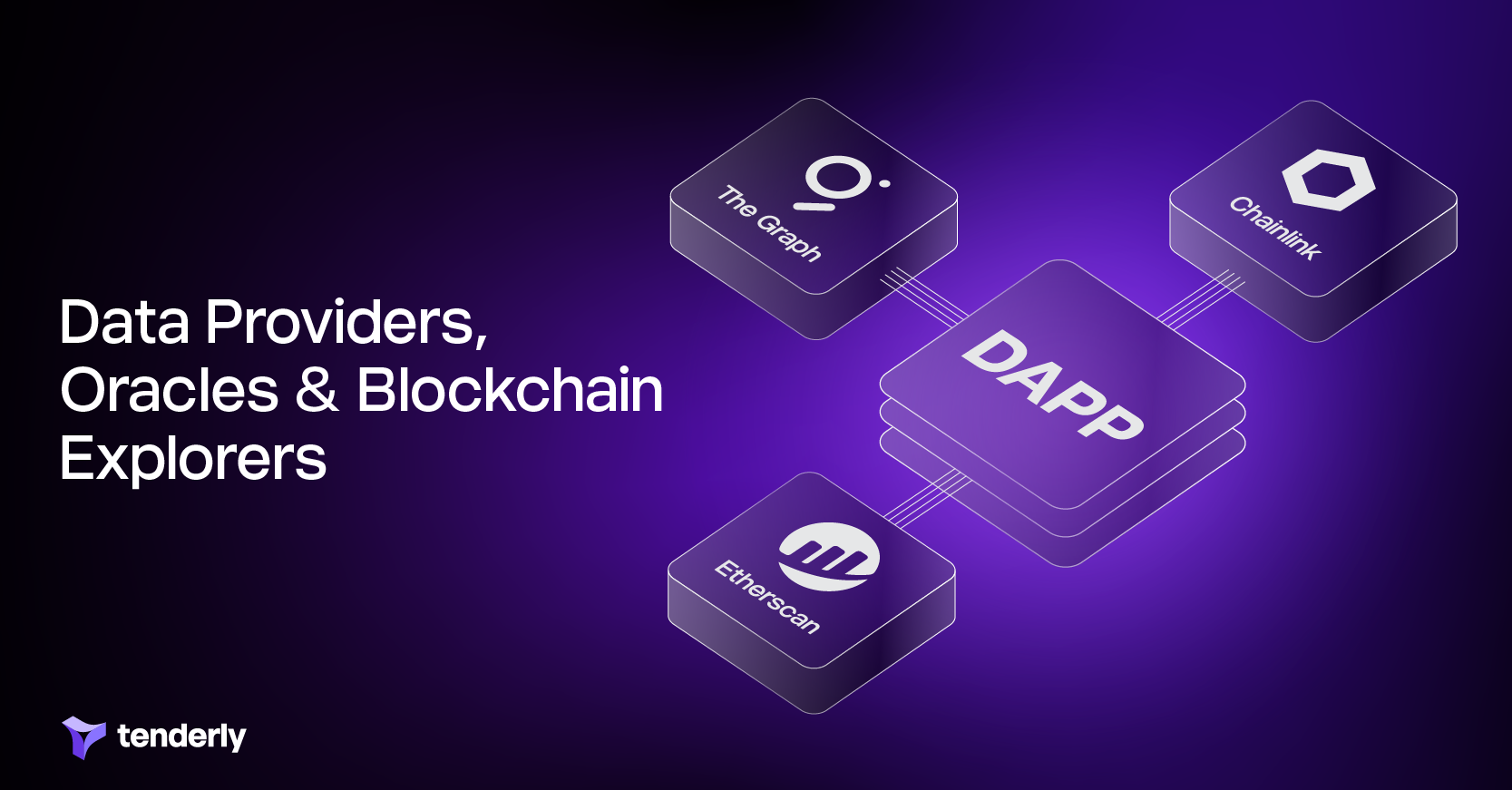
In the context of a web3 tech stack, data providers enable dapps and smart contracts to access, analyze, and use data both from within and outside the blockchain. These providers bridge the gap between on-chain and off-chain worlds, enabling richer functionalities and deeper insights.
Here's a breakdown of essential data providers in the web3 ecosystem:
- Block explorers: Tools that provide insights into transactions and the overall state of the blockchain. Blockchain explorers like Etherscan or open-srouce Blockscout offer a comprehensive view of transaction histories, contract interactions, and token transfers.
- Oracles: Smart contracts often need real-world data, be it price feeds, weather updates, or any external information. Oracles act as trusted data feeds that relay off-chain data to the blockchain. Chainlink is a prominent oracle provider for Ethereum developers.
- The Graph: A decentralized protocol for indexing blockchain data. The Graph allows you to craft and publish open APIs, called subgraphs. This makes data retrieval more efficient and decentralized, ensuring that dapps can query data in a scalable and reliable manner.
Analytics and monitoring
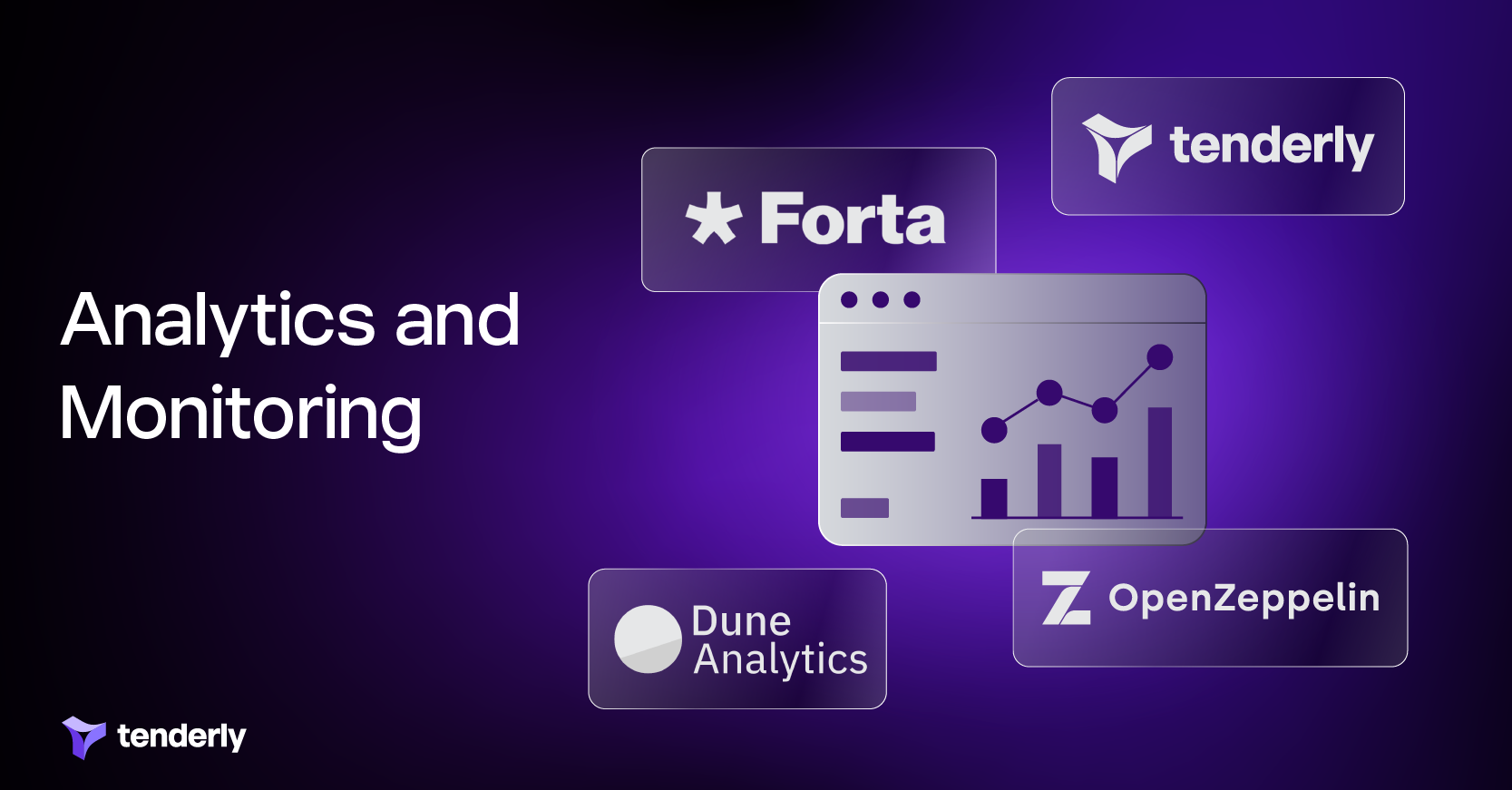
The Ethereum blockchain generates a lot of data through every transaction and contract interaction. Various analytics and monitoring tools have been developed to interpret this data.
These tools analyze transaction patterns, smart contract interactions, and network health, giving you valuable insights for informed decision-making.
Dune Analytics
Dune Analytics offers a user-friendly platform for exploring and sharing blockchain data. Users can easily access analytics for various DeFi projects, dapps, and NFTs through pre-made dashboards. The platform also allows you to create your own data dashboards for your web3 projects. You can run custom SQL queries to extract cross-chain data for analytics purposes for different tokens, wallets, and protocols.
Tenderly Alerts
Tenderly offers a wide range of monitoring and alerting tools for Ethereum projects. Tenderly’s Alerting platform can send you detailed notifications when a specific event happens on your wallet or contracts. You can go granular with monitoring state changes or function calls for specific contracts. When something happens, Tenderly sends you a notification via Email, Slack, or Telegram. Alert data can also be sent to a webhook or a third-party monitoring system.
Tenderly offers 12 robust alert types or events that you can monitor. For example, you can monitor all failed transactions on your contract deployed on the Mainnet, Polygon, or any one of the 30+ supported networks.
Take a look at this quick video demo to learn how to configure and activate alerts with Tenderly.
Forta
Forta monitors on-chain activity in real-time to identify and alert about potential threats and important events. Forta achieves this through a vast network of detection bots. These bots act like security cameras monitoring something specific on-chain. For example, you can use Forta to detect scams, spam, rug-pull attacks, or suspicious activity before funds are stolen.
OpenZeppelin Defender
OpenZeppelin Defender helps you proactively monitor and react to events, functions, and transaction parameters in your smart contracts. Defender also enables you to implement automated actions that enable your team to respond to threats instantly and receive immediate notifications through email, Slack, Telegram, or Discord.
Decentralized storage
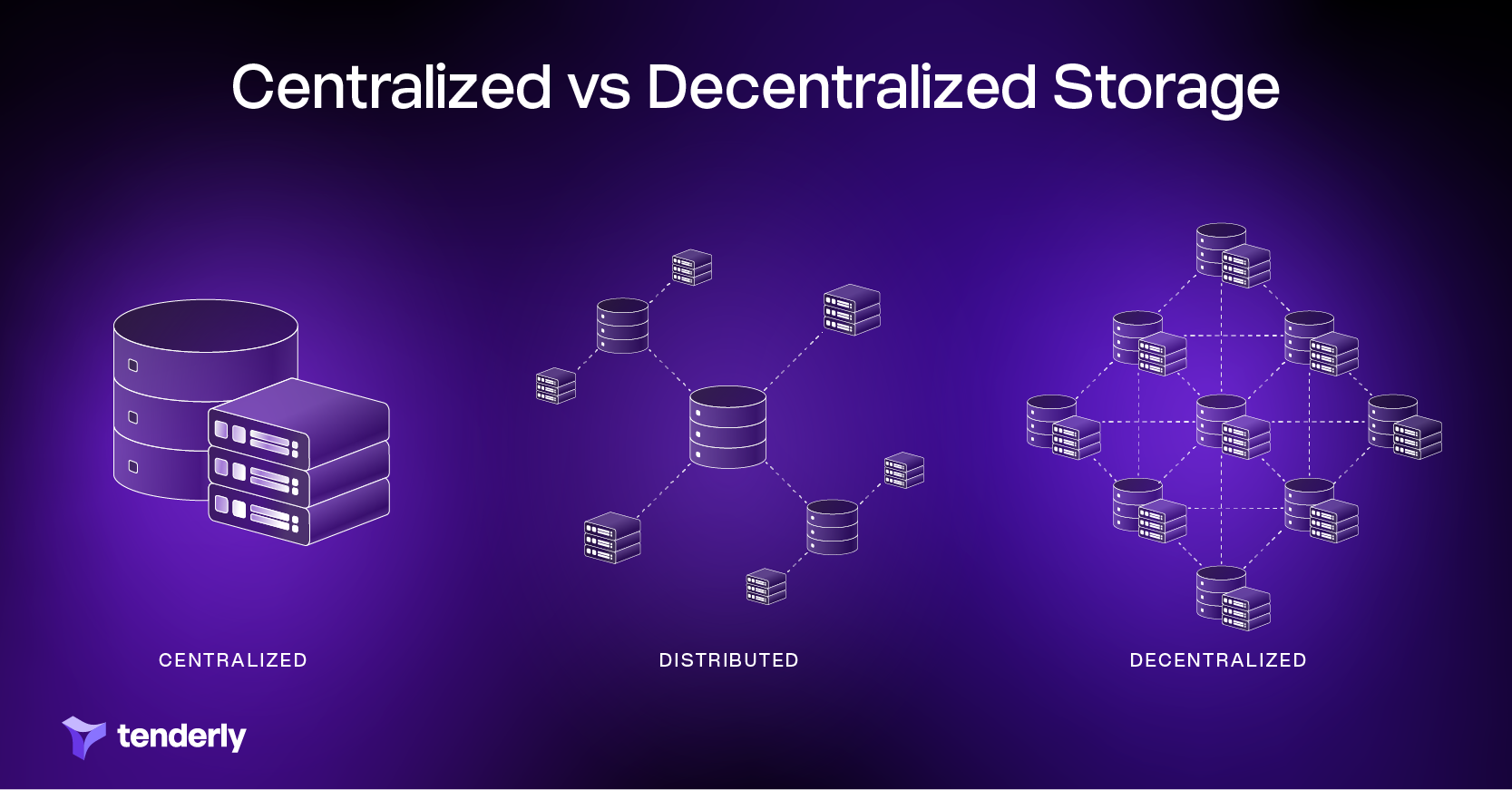
Decentralized storage distributes data across a network of nodes, ensuring redundancy, security, and censorship resistance. This is in contrast to centralized storage, where data is stored on a single server or a cluster of servers owned by one entity.
Here are some of the most popular decentralized storage systems for Ethereum:
- IPFS (InterPlanetary File System): Peer-to-peer protocol that allows users to store and share files in a decentralized manner. It uses content-addressing to ensure that files are uniquely identified and can be accessed from any node on the network.
- Filecoin: A decentralized storage network that allows users to store, retrieve, and share data. It uses a proof-of-replication and proof-of-spacetime consensus mechanism to ensure that data is stored securely and efficiently.
- Arweave: A decentralized storage platform that enables permanent data storage on the decentralized web inside an open ledger. Arweave uses a blockchain-based consensus algorithm called Proof-of-Access. Arweave created its own digital currency called an AR token, which is used to pay for data storage on the permaweb.
What does your web3 tech stack look like?
Experience the difference that Tenderly can make in your web3 development process. Dive into a world where each tool is designed with your needs in mind, ensuring a smoother, more efficient pathway from development to deployment.
Try Tenderly now and push your projects to production faster without the hassle of managing infrastructure or developing your own tools.